Microsoft Edge
To embed Microsoft Edge into our container window we use the WebView2 control. Unfortunately,
embedding Edge will not work
As a side note, I must say that I found it difficult to find good documentation from Microsoft about the steps that needed to be taken to get this to work and, as a result, this was a process with more pain than was reasonable given the small amount of code involved.
Prerequisites
To build and run the example, the following must be installed:
- Microsoft Edge WebView2 Evergreen Runtime.
- Microsoft.Web.WebView2 NuGet package in Visual Studio.
- Microsoft.Windows.ImplementationLibrary NuGet package in Visual Studio.
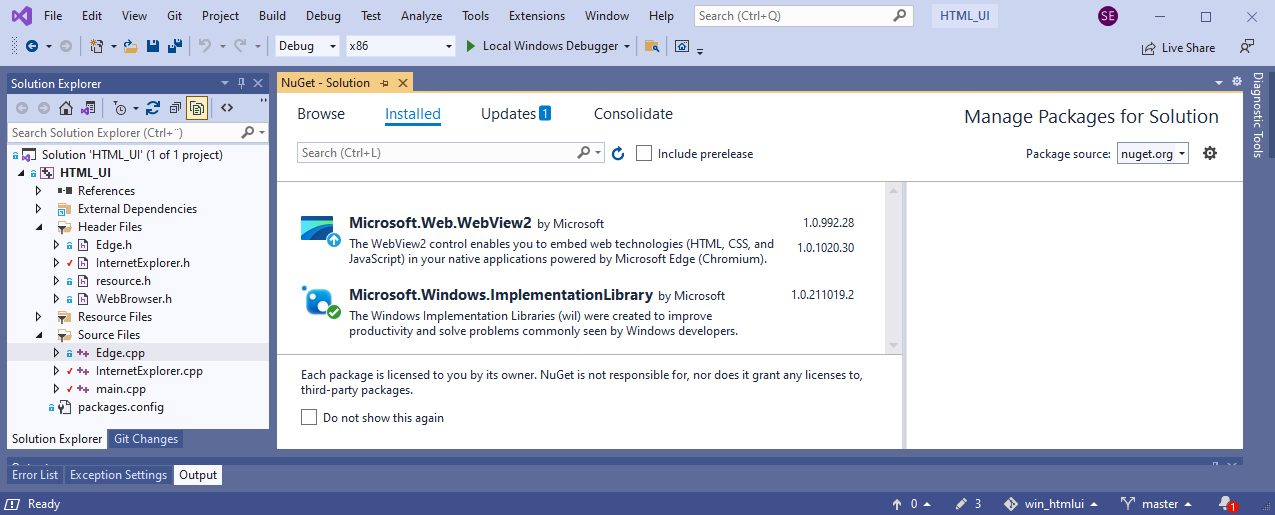
Creating the Browser Control
The browser control is created asynchronously by first creating a WebView2 environment and then creating a WebView2 controller, from which a WebView2 window can be obtained.
The method to create the environment, CreateCoreWebView2EnvironmentWithOptions, is the most fraught with danger. It is this function which attempts to find the Edge WebView2 runtime and if it fails, it will return ERROR_FILE_NOT_FOUND. I just check whether it succeeded or not, but you may want to check for that specific return value.
Assuming all went well, the CEdge::OnCreateEnvironmentCompleted callback will be invoked, which takes the environment instance and calls CreateCoreWebView2Controller, passing another function to be called on success. That callback is where we get the WebView2 window to allow us to implement the Navigate and Refresh functions.
Navigation
Navigation in the CEdge class has to work slightly differently to the way it works in the Internet Explorer implementation. The issue is that the message loop must be running before we can get the WebView2 window, which implements the Navigate function. The main program calls CWebBrowser::Navigate to set the URL before we enter the message loop. To solve this, CEdge::Navigate checks whether the WebView2 window is available and, if not, it assumes that OnCreateCoreWebView2ControllerCompleted has not been called and stores the URL for later use in that function. If the WebView2 window has been created, then we call its Navigate function immediately.
Refresh and RunMessageLoop Functions
The Refresh and RunMessageLoop functions are trivially implemented. Refresh is simply a wrapper
for the WebView2 window Reload function and RunMessageLoop implements textbook Windows message
loop code without the need for any
If everything works, you should see something like this.